C# polymorphism
Polymorphism is the ability of the same behavior to have many different forms or forms of expression.
Polymorphism means multiple forms. In the object-oriented programming paradigm, polymorphism is often expressed as “one interface, multiple functions”.
Polymorphism can be static or dynamic. In static polymorphism, the response of a function occurs at compile time. In dynamic polymorphism, the response of a function occurs at run time.
In C #, every type is polymorphic because all types, including user-defined types, inherit from Object.
Polymorphism is the same interface that uses different instances to perform different operations, as shown in the figure:
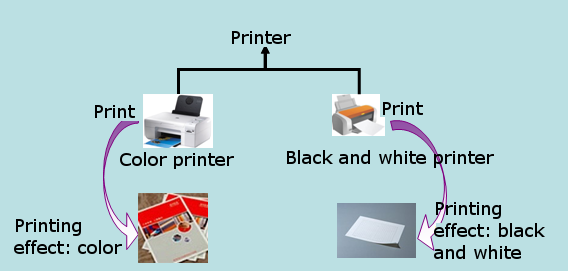
In reality, for example, we press the F1 key:
If what currently pops up under the Flash interface is the help document forAS 3
If the current pop-up under Word is Word help
What pops up under Windows is Windows help and support.
The same event occurs on different objects and produces different results.
Static polymorphism
At compile time, the connection mechanism between functions and objects is called early binding, also known as static binding. C# provides two techniques to achieve static polymorphism. They are:
Function overload
Operator overloading
Operator overloading is discussed in the next section, and we will discuss function overloading next.
Function overload
You can have multiple definitions of the same function name within the same scope. The definitions of functions must be different from each other, either the type of parameters in the parameter list or the number of parameters. Only function declarations with different return types cannot beoverloaded.
The following example demonstrates several of the same functions Add()
used to add different number of parameters:
Example
using System;
namespace PolymorphismApplication
{
public class TestData
{
public int Add(int a, int b, int c)
{
return a + b + c;
}
public int Add(int a, int b)
{
return a + b;
}
}
class Program
{
static void Main(string[] args)
{
TestData dataClass = new TestData();
int add1 = dataClass.Add(1, 2);
int add2 = dataClass.Add(1, 2, 3);
Console.WriteLine("add1 :" + add1);
Console.WriteLine("add2 :" + add2);
}
}
}
The following example demonstrates several of the same functions print()
for printing different data types
Example
using System;
namespace PolymorphismApplication
{
class Printdata
{
void print(int i)
{
Console.WriteLine("Output integer: {0}", i );
}
void print(double f)
{
Console.WriteLine("Output Floating Point: {0}" , f);
}
void print(string s)
{
Console.WriteLine("String: {0}", s);
}
static void Main(string[] args)
{
Printdata p = new Printdata();
// Calling print to print integers
p.print(1);
// Calling print to print floating-point numbers
p.print(1.23);
// Calling print to print a string
p.print("Hello Runoob");
Console.ReadKey();
}
}
}
When the above code is compiled and executed, it produces the following results:
Output integer: 1
Output floating-point type: 1.23
Output string: Hello Runoob
Dynamic polymorphism
C # allows you to use keywords abstract
create an abstract class that provides the implementation of some of the classes that provide the interface. When a derived class inherits from the abstract class, the implementation is complete. Abstract classes contain abstract methods, whichcan be implemented by derived classes. Derived classes have more professional functionality.
Note that here are some rules for abstract classes:
You cannot create an instance of an abstract class.
You cannot declare an abstract method outside an abstract class.
By placing keywords in front of the class definition
sealed
, you can declare a class as a sealed class When a class is declared assealed
,which cannot be inherited. An abstract class cannot be declared assealed
.
The following program demonstrates an abstract class:
Example
using System;
namespace PolymorphismApplication
{
abstract class Shape
{
abstract public int area();
}
class Rectangle: Shape
{
private int length;
private int width;
public Rectangle( int a=0, int b=0)
{
length = a;
width = b;
}
public override int area ()
{
Console.WriteLine("Area of the Rectangle class:");
return (width * length);
}
}
class RectangleTester
{
static void Main(string[] args)
{
Rectangle r = new Rectangle(10, 7);
double a = r.area();
Console.WriteLine("Area: {0}",a);
Console.ReadKey();
}
}
}
When the above code is compiled and executed, it produces the following results:
Area of Rectangle class:
Area: 70
You can use virtual methods when there is a function defined in a class that needs to be implemented in an inherited class.
The virtual method is to use keywords virtual
declared.
Virtual methods can have different implementations in different inherited classes.
Calls to virtual methods occur at run time.
Dynamic polymorphism is achieved through abstract classes and virtual methods.
The following example creates the Shape
base classand creates derived classes such as Circle
, Rectangle
,and Triangle
. The Shape
class provides a virtualmethod called Draw
, which is rewritten in each derivedclass to draw the specified shape of the class.
Example
using System;
using System.Collections.Generic;
public class Shape
{
public int X { get; private set; }
public int Y { get; private set; }
public int Height { get; set; }
public int Width { get; set; }
// Virtual method
public virtual void Draw()
{
Console.WriteLine("Execute drawing tasks for base classes");
}
}
class Circle : Shape
{
public override void Draw()
{
Console.WriteLine("Draw a circle");
base.Draw();
}
}
class Rectangle : Shape
{
public override void Draw()
{
Console.WriteLine("draw a rectangle");
base.Draw();
}
}
class Triangle : Shape
{
public override void Draw()
{
Console.WriteLine("draw a triangle");
base.Draw();
}
}
class Program
{
static void Main(string[] args)
{
// Create a List<Shape>object and add Circle,
Triangle, and Rectangle to it
var shapes = new List<Shape>
{
new Rectangle(),
new Triangle(),
new Circle()
};
// Use a foreach loop to iterate over the derived
classes of the list and call the Draw method on each Shape
object within it
foreach (var shape in shapes)
{
shape.Draw();
}
Console.WriteLine("Press any key to exit。");
Console.ReadKey();
}
}
When the above code is compiled and executed, it produces the following results:
Draw a rectangle
Execute drawing tasks for base classes
Draw a triangle
Execute drawing tasks for base classes
Draw a circle
Execute drawing tasks for base classes
Press any key to exit.
The following program demonstrates how to use the virtual method area()
to calculate the area of images of different shapes:
Example
using System;
namespace PolymorphismApplication
{
class Shape
{
protected int width, height;
public Shape( int a=0, int b=0)
{
width = a;
height = b;
}
public virtual int area()
{
Console.WriteLine("Area of parent class:");
return 0;
}
}
class Rectangle: Shape
{
public Rectangle( int a=0, int b=0): base(a, b)
{
}
public override int area ()
{
Console.WriteLine("Area of the Rectangle class:");
return (width * height);
}
}
class Triangle: Shape
{
public Triangle(int a = 0, int b = 0): base(a, b)
{
}
public override int area()
{
Console.WriteLine("Area of the Triangle class:");
return (width * height / 2);
}
}
class Caller
{
public void CallArea(Shape sh)
{
int a;
a = sh.area();
Console.WriteLine("Area: {0}", a);
}
}
class Tester
{
static void Main(string[] args)
{
Caller c = new Caller();
Rectangle r = new Rectangle(10, 7);
Triangle t = new Triangle(10, 5);
c.CallArea(r);
c.CallArea(t);
Console.ReadKey();
}
}
}
When the above code is compiled and executed, it produces the following results:
Area of Rectangle class:
Area: 70
Area of Triangle class:
Area: 25